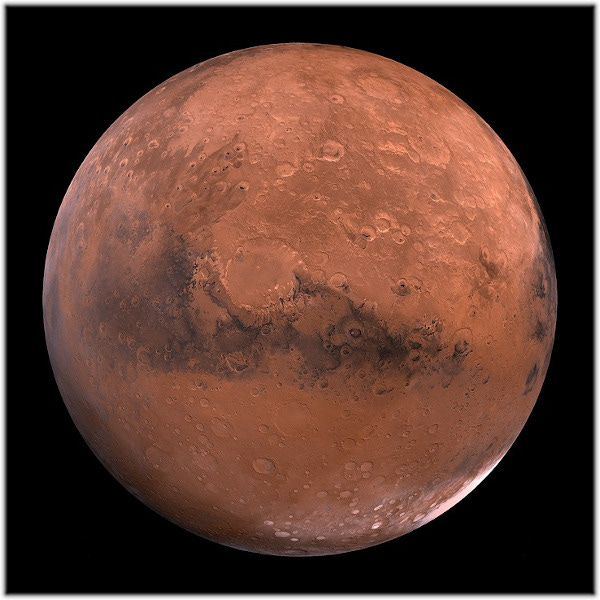
In 1687 Isaac Newton published PhilosophiƦ Naturalis Principia Mathematica or Mathematical Principles of Natural Philosophy. It is one of the most important and profound scientific works ever written and includes Newton's Law of Universal Gravitation. This law states that all matter exerts a gravitational attraction on all other matter, and provides a simple formula describing that attraction.
In this post I will use that formula to calculate how much a person would weigh if they were standing on Mars.
This is the formula:
and it tells us that if we know the mass of the two objects we are interested in (for example a planet and a person) and the distance between their centres of mass we can work out the force each exerts on the other. The individual terms are:
F - the force in Newtons each body exerts on the other
G - the universal gravitational constant, quite literally constant throughout the universe
m1 and m2 - the masses of the two bodies we are interested in
r - the distance between the centres of mass of the two bodies. Note that this is squared.
I will be using kilograms and metres for masses and distances respectively. However, other units with the same proportions can be used, for example when dealing with two planets it might be more convenient to use tonnes and kilometres, each of which is 1000 times larger than kilograms and metres.
It's important to realise that the formula works in both directions, ie it tells us how much force a planet exerts on a person AND how much force that person exerts on the planet. The two are identical. This can be difficult to comprehend until you realise that the two masses multiplied together are then divided by the distance r SQUARED, therefore the decrease in the force of gravity with distance decreases according to the inverse square law. The bits of the planet 12 feet away from you only exert 25% of the force as those bits 6 feet away, those 24 feet away only exert 12.5% of the force and so on. And even on a small planet like Earth a part of the planet is about 8000 miles away and that part only exerts about one seven millionth as much force as the parts 6 feet away.
This project consists of a single file called martiangravity.py which you can clone/download from the Github repository.
This is the code listing in its entirety.
from typing import Dict
def main():
print("-------------------")
print("| codedrome.com |")
print("| Martian Gravity |")
print("-------------------\n")
planet = "mars"
human_weight_kg = 75 # 165lbs / 11.8st
weights = calculate_weights(planet, human_weight_kg)
print(f"A person weighing {human_weight_kg}kg on Earth would weigh:\n")
print(f" {weights['pounds']:>3.2f}lbs")
print(f" {weights['stones']:>2.2f}st")
print(f" {weights['kilograms']:>3.2f}kg")
print(f"\non {planet.capitalize()}.")
def calculate_weights(planet: str, human_weight_kg: float) -> Dict:
"""
An implementation of Newton's
Law of Universal Gravitation.
The name of the planet is used to get
the relevant mass and radius.
These and the Earth weight of the person
are used to calculate the person's
weight on the specified planet.
"""
planet_details = get_planet_details()
# variables used in formula
G = 6.67408 * 10**-11
m1 = planet_details[planet]["mass_kg"]
m2 = human_weight_kg
r = planet_details[planet]["mean_radius_metres"]
# evaluate the formula.
# note: the result is the force in newtons
# which then has to be converted to kg etc.
F = G * ( (m1 * m2) / (r**2 ) )
weights = newtons_to_weights(F)
return weights
def get_planet_details() -> Dict:
"""
Create and return a dictionary of
hard-coded planet data:
masses in kilograms
radii in metres
"""
planet_details = {}
planet_details["mercury"] = {"mass_kg": 3.3011 * 10**23,
"mean_radius_metres": 2439.7 * 1000}
planet_details["venus"] = {"mass_kg": 4.8675 * 10**24,
"mean_radius_metres": 6051.8 * 1000}
planet_details["earth"] = {"mass_kg": 5.97237 * 10**24,
"mean_radius_metres": 6371 * 1000}
planet_details["mars"] = {"mass_kg": 6.4171 * 10**23,
"mean_radius_metres": 3389.5 * 1000}
planet_details["jupiter"] = {"mass_kg": 1.8982 * 10**27,
"mean_radius_metres": 69911 * 1000}
planet_details["saturn"] = {"mass_kg": 5.6834 * 10**26,
"mean_radius_metres": 58232 * 1000}
planet_details["uranus"] = {"mass_kg": 8.681 * 10**25,
"mean_radius_metres": 25362 * 1000}
planet_details["neptune"] = {"mass_kg": 1.02413 * 10**26,
"mean_radius_metres": 24622 * 1000}
return planet_details
def newtons_to_weights(newtons: float) -> Dict:
"""
Convert newtons to various weight units
by division by appropriate amount.
"""
weights = {}
weights["newtons"] = newtons
weights["pounds"] = newtons / 4.4482216
weights["stones"] = weights["pounds"] / 14
weights["kilograms"] = newtons / 9.80665
return weights
if __name__ == "__main__":
main()
main
Here we create a couple of variables for values which are then passed to the calculate_weights function. This returns a dictionary which is printed out in a neat and informative way.
calculate_weights
This function takes as arguments the name of a planet and the weight of a person. Although this project is mainly about Mars it can handle all the other planets including Earth. (But not poor old Pluto, nor yet its possible replacement as the ninth planet...)
Firstly we call a separate function to grab a dictionary of details on the nine planets, and declare a variable G, initialised to the gravitational constant which as you can see is pretty tiny.
The two masses and the radius are stored in separate variables to make the formula, which is implemented next, more concise. As I mentioned the result is the force in Newtons (the SI unit of force) which is fair enough but meaningless to most people so it is passed to newtons_to_weights which returns the equivalent in several more familiar units, this being returned by the function.
get_planet_details
This is straightforward - it just returns a dictionary of hard-coded weights and radii. There are a couple of things to note here:
Planets aren't usually exactly spherical but slightly flattened due to the centrifugal source of their rotation. (Astronomy books usually say "like a tangerine".) The radius therefore varies so I am using the mean.
Strictly speaking we need the distance between the centres of mass of the two objects. However, the size of a human is so insignificant compared to a planet that just using the planet's radius is fine. (If you are trying to land a probe on a tiny asteroid you would need more precision.)
newtons_to_weights
This is the function I mentioned above which takes a value in Newtons and returns a dictionary containing the original Newton value and its equivalents in pounds, stones and kilograms.
Now let's run the program.
python3 martiangravity.py
The output looks like this:
So if you were to visit the Red Planet you wouldn't weigh much more than a third of your Earth weight, and you might like to edit the name of the planet in main to see how much you'd weigh on the other planets. However, in some cases this is hypothetical: Jupiter and Saturn are gas giants consisting mostly of hydrogen and helium so have no firm surface, Mercury's temperature fluctuates between -170°C and 420°C, and Venus is surrounded by clouds of sulphuric acid. My recommendation to anyone thinking of visiting another planet is to choose Mars!